(PHP 4, PHP 5, PHP 7)
Draws an arc
bool imagearc ( resource $image, int $cx, int $cy, int $width, int $height, int $start, int $end, int $color )
imagearc() draws an arc of circle centered at the given coordinates.
Parameters:
image
An image resource, returned by one of the image creation functions, such as imagecreatetruecolor().
cx
x-coordinate of the center.
cy
y-coordinate of the center.
width
The arc width.
height
The arc height.
start
The arc start angle, in degrees.
end
The arc end angle, in degrees. 0° is located at the three-o'clock position, and the arc is drawn clockwise.
color
A color identifier created with imagecolorallocate().
Returns:
Returns TRUE
on success or FALSE
on failure.
Examples:
Drawing a circle with imagearc()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | <?php // create a 200*200 image $img = imagecreatetruecolor(200, 200); // allocate some colors $white = imagecolorallocate( $img , 255, 255, 255); $red = imagecolorallocate( $img , 255, 0, 0); $green = imagecolorallocate( $img , 0, 255, 0); $blue = imagecolorallocate( $img , 0, 0, 255); // draw the head imagearc( $img , 100, 100, 200, 200, 0, 360, $white ); // mouth imagearc( $img , 100, 100, 150, 150, 25, 155, $red ); // left and then the right eye imagearc( $img , 60, 75, 50, 50, 0, 360, $green ); imagearc( $img , 140, 75, 50, 50, 0, 360, $blue ); // output image in the browser header( "Content-type: image/png" ); imagepng( $img ); // free memory imagedestroy( $img ); ?> |
The above example will output something similar to:
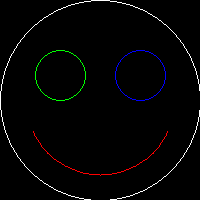
See also:
Please login to continue.