-
numpy.broadcast_arrays(*args, **kwargs)
[source] -
Broadcast any number of arrays against each other.
Parameters: `*args` : array_likes
The arrays to broadcast.
subok : bool, optional
If True, then sub-classes will be passed-through, otherwise the returned arrays will be forced to be a base-class array (default).
Returns: broadcasted : list of arrays
These arrays are views on the original arrays. They are typically not contiguous. Furthermore, more than one element of a broadcasted array may refer to a single memory location. If you need to write to the arrays, make copies first.
Examples
>>> x = np.array([[1,2,3]]) >>> y = np.array([[1],[2],[3]]) >>> np.broadcast_arrays(x, y) [array([[1, 2, 3], [1, 2, 3], [1, 2, 3]]), array([[1, 1, 1], [2, 2, 2], [3, 3, 3]])]
Here is a useful idiom for getting contiguous copies instead of non-contiguous views.
>>> [np.array(a) for a in np.broadcast_arrays(x, y)] [array([[1, 2, 3], [1, 2, 3], [1, 2, 3]]), array([[1, 1, 1], [2, 2, 2], [3, 3, 3]])]
numpy.broadcast_arrays()
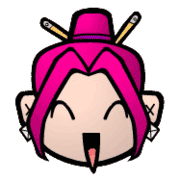
2017-01-10 18:13:00
Please login to continue.