-
numpy.fill_diagonal(a, val, wrap=False)
[source] -
Fill the main diagonal of the given array of any dimensionality.
For an array
a
witha.ndim > 2
, the diagonal is the list of locations with indicesa[i, i, ..., i]
all identical. This function modifies the input array in-place, it does not return a value.Parameters: a : array, at least 2-D.
Array whose diagonal is to be filled, it gets modified in-place.
val : scalar
Value to be written on the diagonal, its type must be compatible with that of the array a.
wrap : bool
For tall matrices in NumPy version up to 1.6.2, the diagonal ?wrapped? after N columns. You can have this behavior with this option. This affects only tall matrices.
See also
Notes
New in version 1.4.0.
This functionality can be obtained via
diag_indices
, but internally this version uses a much faster implementation that never constructs the indices and uses simple slicing.Examples
>>> a = np.zeros((3, 3), int) >>> np.fill_diagonal(a, 5) >>> a array([[5, 0, 0], [0, 5, 0], [0, 0, 5]])
The same function can operate on a 4-D array:
>>> a = np.zeros((3, 3, 3, 3), int) >>> np.fill_diagonal(a, 4)
We only show a few blocks for clarity:
>>> a[0, 0] array([[4, 0, 0], [0, 0, 0], [0, 0, 0]]) >>> a[1, 1] array([[0, 0, 0], [0, 4, 0], [0, 0, 0]]) >>> a[2, 2] array([[0, 0, 0], [0, 0, 0], [0, 0, 4]])
The wrap option affects only tall matrices:
>>> # tall matrices no wrap >>> a = np.zeros((5, 3),int) >>> fill_diagonal(a, 4) >>> a array([[4, 0, 0], [0, 4, 0], [0, 0, 4], [0, 0, 0], [0, 0, 0]])
>>> # tall matrices wrap >>> a = np.zeros((5, 3),int) >>> fill_diagonal(a, 4, wrap=True) >>> a array([[4, 0, 0], [0, 4, 0], [0, 0, 4], [0, 0, 0], [4, 0, 0]])
>>> # wide matrices >>> a = np.zeros((3, 5),int) >>> fill_diagonal(a, 4, wrap=True) >>> a array([[4, 0, 0, 0, 0], [0, 4, 0, 0, 0], [0, 0, 4, 0, 0]])
numpy.fill_diagonal()
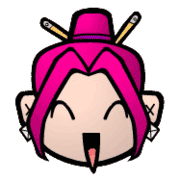
2017-01-10 18:14:00
Please login to continue.