-
numpy.tensordot(a, b, axes=2)
[source] -
Compute tensor dot product along specified axes for arrays >= 1-D.
Given two tensors (arrays of dimension greater than or equal to one),
a
andb
, and an array_like object containing two array_like objects,(a_axes, b_axes)
, sum the products ofa
?s andb
?s elements (components) over the axes specified bya_axes
andb_axes
. The third argument can be a single non-negative integer_like scalar,N
; if it is such, then the lastN
dimensions ofa
and the firstN
dimensions ofb
are summed over.Parameters: a, b : array_like, len(shape) >= 1
Tensors to ?dot?.
axes : int or (2,) array_like
- integer_like If an int N, sum over the last N axes of
a
and the first N axes ofb
in order. The sizes of the corresponding axes must match. - (2,) array_like Or, a list of axes to be summed over, first sequence applying to
a
, second tob
. Both elements array_like must be of the same length.
Notes
- Three common use cases are:
-
axes = 0
: tensor product $aotimes b$axes = 1
: tensor dot product $acdot b$axes = 2
: (default) tensor double contraction $a:b$
When
axes
is integer_like, the sequence for evaluation will be: first the -Nth axis ina
and 0th axis inb
, and the -1th axis ina
and Nth axis inb
last.When there is more than one axis to sum over - and they are not the last (first) axes of
a
(b
) - the argumentaxes
should consist of two sequences of the same length, with the first axis to sum over given first in both sequences, the second axis second, and so forth.Examples
A ?traditional? example:
123456789101112131415161718192021222324>>> a
=
np.arange(
60.
).reshape(
3
,
4
,
5
)
>>> b
=
np.arange(
24.
).reshape(
4
,
3
,
2
)
>>> c
=
np.tensordot(a,b, axes
=
([
1
,
0
],[
0
,
1
]))
>>> c.shape
(
5
,
2
)
>>> c
array([[
4400.
,
4730.
],
[
4532.
,
4874.
],
[
4664.
,
5018.
],
[
4796.
,
5162.
],
[
4928.
,
5306.
]])
>>>
# A slower but equivalent way of computing the same...
>>> d
=
np.zeros((
5
,
2
))
>>>
for
i
in
range
(
5
):
...
for
j
in
range
(
2
):
...
for
k
in
range
(
3
):
...
for
n
in
range
(
4
):
... d[i,j]
+
=
a[k,n,i]
*
b[n,k,j]
>>> c
=
=
d
array([[
True
,
True
],
[
True
,
True
],
[
True
,
True
],
[
True
,
True
],
[
True
,
True
]], dtype
=
bool
)
An extended example taking advantage of the overloading of + and *:
1234567891011>>> a
=
np.array(
range
(
1
,
9
))
>>> a.shape
=
(
2
,
2
,
2
)
>>> A
=
np.array((
'a'
,
'b'
,
'c'
,
'd'
), dtype
=
object
)
>>> A.shape
=
(
2
,
2
)
>>> a; A
array([[[
1
,
2
],
[
3
,
4
]],
[[
5
,
6
],
[
7
,
8
]]])
array([[a, b],
[c, d]], dtype
=
object
)
12>>> np.tensordot(a, A)
# third argument default is 2 for double-contraction
array([abbcccdddd, aaaaabbbbbbcccccccdddddddd], dtype
=
object
)
12345>>> np.tensordot(a, A,
1
)
array([[[acc, bdd],
[aaacccc, bbbdddd]],
[[aaaaacccccc, bbbbbdddddd],
[aaaaaaacccccccc, bbbbbbbdddddddd]]], dtype
=
object
)
1234>>> np.tensordot(a, A,
0
)
# tensor product (result too long to incl.)
array([[[[[a, b],
[c, d]],
...
12345>>> np.tensordot(a, A, (
0
,
1
))
array([[[abbbbb, cddddd],
[aabbbbbb, ccdddddd]],
[[aaabbbbbbb, cccddddddd],
[aaaabbbbbbbb, ccccdddddddd]]], dtype
=
object
)
12345>>> np.tensordot(a, A, (
2
,
1
))
array([[[abb, cdd],
[aaabbbb, cccdddd]],
[[aaaaabbbbbb, cccccdddddd],
[aaaaaaabbbbbbbb, cccccccdddddddd]]], dtype
=
object
)
12>>> np.tensordot(a, A, ((
0
,
1
), (
0
,
1
)))
array([abbbcccccddddddd, aabbbbccccccdddddddd], dtype
=
object
)
12>>> np.tensordot(a, A, ((
2
,
1
), (
1
,
0
)))
array([acccbbdddd, aaaaacccccccbbbbbbdddddddd], dtype
=
object
)
- integer_like If an int N, sum over the last N axes of
numpy.tensordot()
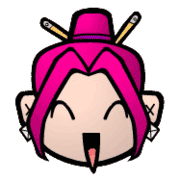
2025-01-10 15:47:30
Please login to continue.