-
numpy.divide(x1, x2[, out]) =
-
Divide arguments element-wise.
Parameters: x1 : array_like
Dividend array.
x2 : array_like
Divisor array.
out : ndarray, optional
Array into which the output is placed. Its type is preserved and it must be of the right shape to hold the output. See doc.ufuncs.
Returns: y : ndarray or scalar
The quotient
x1/x2
, element-wise. Returns a scalar if bothx1
andx2
are scalars.See also
-
seterr
- Set whether to raise or warn on overflow, underflow and division by zero.
Notes
Equivalent to
x1
/x2
in terms of array-broadcasting.Behavior on division by zero can be changed using
seterr
.In Python 2, when both
x1
andx2
are of an integer type,divide
will behave likefloor_divide
. In Python 3, it behaves liketrue_divide
.Examples
>>> np.divide(2.0, 4.0) 0.5 >>> x1 = np.arange(9.0).reshape((3, 3)) >>> x2 = np.arange(3.0) >>> np.divide(x1, x2) array([[ NaN, 1. , 1. ], [ Inf, 4. , 2.5], [ Inf, 7. , 4. ]])
Note the behavior with integer types (Python 2 only):
>>> np.divide(2, 4) 0 >>> np.divide(2, 4.) 0.5
Division by zero always yields zero in integer arithmetic (again, Python 2 only), and does not raise an exception or a warning:
>>> np.divide(np.array([0, 1], dtype=int), np.array([0, 0], dtype=int)) array([0, 0])
Division by zero can, however, be caught using
seterr
:>>> old_err_state = np.seterr(divide='raise') >>> np.divide(1, 0) Traceback (most recent call last): File "<stdin>", line 1, in <module> FloatingPointError: divide by zero encountered in divide
>>> ignored_states = np.seterr(**old_err_state) >>> np.divide(1, 0) 0
-
numpy.divide()
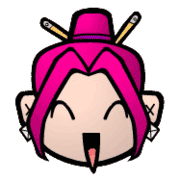
2017-01-10 18:13:42
Please login to continue.