-
MaskedArray.nonzero()
[source] -
Return the indices of unmasked elements that are not zero.
Returns a tuple of arrays, one for each dimension, containing the indices of the non-zero elements in that dimension. The corresponding non-zero values can be obtained with:
a[a.nonzero()]
To group the indices by element, rather than dimension, use instead:
np.transpose(a.nonzero())
The result of this is always a 2d array, with a row for each non-zero element.
Parameters: None
Returns: tuple_of_arrays : tuple
Indices of elements that are non-zero.
See also
-
numpy.nonzero
- Function operating on ndarrays.
-
flatnonzero
- Return indices that are non-zero in the flattened version of the input array.
-
ndarray.nonzero
- Equivalent ndarray method.
-
count_nonzero
- Counts the number of non-zero elements in the input array.
Examples
>>> import numpy.ma as ma >>> x = ma.array(np.eye(3)) >>> x masked_array(data = [[ 1. 0. 0.] [ 0. 1. 0.] [ 0. 0. 1.]], mask = False, fill_value=1e+20) >>> x.nonzero() (array([0, 1, 2]), array([0, 1, 2]))
Masked elements are ignored.
>>> x[1, 1] = ma.masked >>> x masked_array(data = [[1.0 0.0 0.0] [0.0 -- 0.0] [0.0 0.0 1.0]], mask = [[False False False] [False True False] [False False False]], fill_value=1e+20) >>> x.nonzero() (array([0, 2]), array([0, 2]))
Indices can also be grouped by element.
>>> np.transpose(x.nonzero()) array([[0, 0], [2, 2]])
A common use for
nonzero
is to find the indices of an array, where a condition is True. Given an arraya
, the conditiona
> 3 is a boolean array and since False is interpreted as 0, ma.nonzero(a > 3) yields the indices of thea
where the condition is true.>>> a = ma.array([[1,2,3],[4,5,6],[7,8,9]]) >>> a > 3 masked_array(data = [[False False False] [ True True True] [ True True True]], mask = False, fill_value=999999) >>> ma.nonzero(a > 3) (array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
The
nonzero
method of the condition array can also be called.>>> (a > 3).nonzero() (array([1, 1, 1, 2, 2, 2]), array([0, 1, 2, 0, 1, 2]))
-
MaskedArray.nonzero()
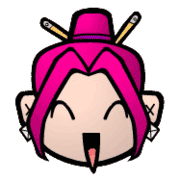
2017-01-10 18:10:14
Please login to continue.