-
numpy.apply_along_axis(func1d, axis, arr, *args, **kwargs)
[source] -
Apply a function to 1-D slices along the given axis.
Execute
func1d(a, *args)
wherefunc1d
operates on 1-D arrays anda
is a 1-D slice ofarr
alongaxis
.Parameters: func1d : function
This function should accept 1-D arrays. It is applied to 1-D slices of
arr
along the specified axis.axis : integer
Axis along which
arr
is sliced.arr : ndarray
Input array.
args : any
Additional arguments to
func1d
.kwargs: any
Additional named arguments to
func1d
.New in version 1.9.0.
Returns: apply_along_axis : ndarray
The output array. The shape of
outarr
is identical to the shape ofarr
, except along theaxis
dimension, where the length ofoutarr
is equal to the size of the return value offunc1d
. Iffunc1d
returns a scalaroutarr
will have one fewer dimensions thanarr
.See also
-
apply_over_axes
- Apply a function repeatedly over multiple axes.
Examples
>>> def my_func(a): ... """Average first and last element of a 1-D array""" ... return (a[0] + a[-1]) * 0.5 >>> b = np.array([[1,2,3], [4,5,6], [7,8,9]]) >>> np.apply_along_axis(my_func, 0, b) array([ 4., 5., 6.]) >>> np.apply_along_axis(my_func, 1, b) array([ 2., 5., 8.])
For a function that doesn?t return a scalar, the number of dimensions in
outarr
is the same asarr
.>>> b = np.array([[8,1,7], [4,3,9], [5,2,6]]) >>> np.apply_along_axis(sorted, 1, b) array([[1, 7, 8], [3, 4, 9], [2, 5, 6]])
-
numpy.apply_along_axis()
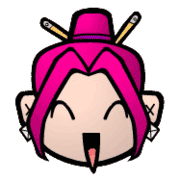
2017-01-10 18:12:38
Please login to continue.