-
class sklearn.cluster.bicluster.SpectralCoclustering(n_clusters=3, svd_method='randomized', n_svd_vecs=None, mini_batch=False, init='k-means++', n_init=10, n_jobs=1, random_state=None)
[source] -
Spectral Co-Clustering algorithm (Dhillon, 2001).
Clusters rows and columns of an array
X
to solve the relaxed normalized cut of the bipartite graph created fromX
as follows: the edge between row vertexi
and column vertexj
has weightX[i, j]
.The resulting bicluster structure is block-diagonal, since each row and each column belongs to exactly one bicluster.
Supports sparse matrices, as long as they are nonnegative.
Read more in the User Guide.
Parameters: n_clusters : integer, optional, default: 3
The number of biclusters to find.
svd_method : string, optional, default: ?randomized?
Selects the algorithm for finding singular vectors. May be ?randomized? or ?arpack?. If ?randomized?, use
sklearn.utils.extmath.randomized_svd
, which may be faster for large matrices. If ?arpack?, usesklearn.utils.arpack.svds
, which is more accurate, but possibly slower in some cases.n_svd_vecs : int, optional, default: None
Number of vectors to use in calculating the SVD. Corresponds to
ncv
whensvd_method=arpack
andn_oversamples
whensvd_method
is ?randomized`.mini_batch : bool, optional, default: False
Whether to use mini-batch k-means, which is faster but may get different results.
init : {?k-means++?, ?random? or an ndarray}
Method for initialization of k-means algorithm; defaults to ?k-means++?.
n_init : int, optional, default: 10
Number of random initializations that are tried with the k-means algorithm.
If mini-batch k-means is used, the best initialization is chosen and the algorithm runs once. Otherwise, the algorithm is run for each initialization and the best solution chosen.
n_jobs : int, optional, default: 1
The number of jobs to use for the computation. This works by breaking down the pairwise matrix into n_jobs even slices and computing them in parallel.
If -1 all CPUs are used. If 1 is given, no parallel computing code is used at all, which is useful for debugging. For n_jobs below -1, (n_cpus + 1 + n_jobs) are used. Thus for n_jobs = -2, all CPUs but one are used.
random_state : int seed, RandomState instance, or None (default)
A pseudo random number generator used by the K-Means initialization.
Attributes: rows_ : array-like, shape (n_row_clusters, n_rows)
Results of the clustering.
rows[i, r]
is True if clusteri
contains rowr
. Available only after callingfit
.columns_ : array-like, shape (n_column_clusters, n_columns)
Results of the clustering, like
rows
.row_labels_ : array-like, shape (n_rows,)
The bicluster label of each row.
column_labels_ : array-like, shape (n_cols,)
The bicluster label of each column.
References
- Dhillon, Inderjit S, 2001. Co-clustering documents and words using bipartite spectral graph partitioning.
Methods
fit
(X)Creates a biclustering for X. get_indices
(i)Row and column indices of the i?th bicluster. get_params
([deep])Get parameters for this estimator. get_shape
(i)Shape of the i?th bicluster. get_submatrix
(i, data)Returns the submatrix corresponding to bicluster i
.set_params
(\*\*params)Set the parameters of this estimator. -
__init__(n_clusters=3, svd_method='randomized', n_svd_vecs=None, mini_batch=False, init='k-means++', n_init=10, n_jobs=1, random_state=None)
[source]
-
biclusters_
-
Convenient way to get row and column indicators together.
Returns the
rows_
andcolumns_
members.
-
fit(X)
[source] -
Creates a biclustering for X.
Parameters: X : array-like, shape (n_samples, n_features)
-
get_indices(i)
[source] -
Row and column indices of the i?th bicluster.
Only works if
rows_
andcolumns_
attributes exist.Returns: row_ind : np.array, dtype=np.intp
Indices of rows in the dataset that belong to the bicluster.
col_ind : np.array, dtype=np.intp
Indices of columns in the dataset that belong to the bicluster.
-
get_params(deep=True)
[source] -
Get parameters for this estimator.
Parameters: deep : boolean, optional
If True, will return the parameters for this estimator and contained subobjects that are estimators.
Returns: params : mapping of string to any
Parameter names mapped to their values.
-
get_shape(i)
[source] -
Shape of the i?th bicluster.
Returns: shape : (int, int)
Number of rows and columns (resp.) in the bicluster.
-
get_submatrix(i, data)
[source] -
Returns the submatrix corresponding to bicluster
i
.Works with sparse matrices. Only works if
rows_
andcolumns_
attributes exist.
-
set_params(**params)
[source] -
Set the parameters of this estimator.
The method works on simple estimators as well as on nested objects (such as pipelines). The latter have parameters of the form
<component>__<parameter>
so that it?s possible to update each component of a nested object.Returns: self :
cluster.bicluster.SpectralCoclustering()
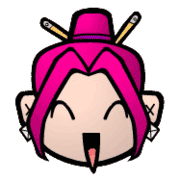
2017-01-15 04:20:39
Please login to continue.