-
numpy.tile(A, reps)
[source] -
Construct an array by repeating A the number of times given by reps.
If
reps
has lengthd
, the result will have dimension ofmax(d, A.ndim)
.If
A.ndim < d
,A
is promoted to be d-dimensional by prepending new axes. So a shape (3,) array is promoted to (1, 3) for 2-D replication, or shape (1, 1, 3) for 3-D replication. If this is not the desired behavior, promoteA
to d-dimensions manually before calling this function.If
A.ndim > d
,reps
is promoted toA
.ndim by pre-pending 1?s to it. Thus for anA
of shape (2, 3, 4, 5), areps
of (2, 2) is treated as (1, 1, 2, 2).Note : Although tile may be used for broadcasting, it is strongly recommended to use numpy?s broadcasting operations and functions.
Parameters: A : array_like
The input array.
reps : array_like
The number of repetitions of
A
along each axis.Returns: c : ndarray
The tiled output array.
See also
-
repeat
- Repeat elements of an array.
-
broadcast_to
- Broadcast an array to a new shape
Examples
>>> a = np.array([0, 1, 2]) >>> np.tile(a, 2) array([0, 1, 2, 0, 1, 2]) >>> np.tile(a, (2, 2)) array([[0, 1, 2, 0, 1, 2], [0, 1, 2, 0, 1, 2]]) >>> np.tile(a, (2, 1, 2)) array([[[0, 1, 2, 0, 1, 2]], [[0, 1, 2, 0, 1, 2]]])
>>> b = np.array([[1, 2], [3, 4]]) >>> np.tile(b, 2) array([[1, 2, 1, 2], [3, 4, 3, 4]]) >>> np.tile(b, (2, 1)) array([[1, 2], [3, 4], [1, 2], [3, 4]])
>>> c = np.array([1,2,3,4]) >>> np.tile(c,(4,1)) array([[1, 2, 3, 4], [1, 2, 3, 4], [1, 2, 3, 4], [1, 2, 3, 4]])
-
numpy.tile()
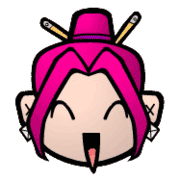
2017-01-10 18:19:01
Please login to continue.