-
numpy.seterrcall(func)
[source] -
Set the floating-point error callback function or log object.
There are two ways to capture floating-point error messages. The first is to set the error-handler to ?call?, using
seterr
. Then, set the function to call using this function.The second is to set the error-handler to ?log?, using
seterr
. Floating-point errors then trigger a call to the ?write? method of the provided object.Parameters: func : callable f(err, flag) or object with write method
Function to call upon floating-point errors (?call?-mode) or object whose ?write? method is used to log such message (?log?-mode).
The call function takes two arguments. The first is a string describing the type of error (such as ?divide by zero?, ?overflow?, ?underflow?, or ?invalid value?), and the second is the status flag. The flag is a byte, whose four least-significant bits indicate the type of error, one of ?divide?, ?over?, ?under?, ?invalid?:
[0 0 0 0 divide over under invalid]
In other words,
flags = divide + 2*over + 4*under + 8*invalid
.If an object is provided, its write method should take one argument, a string.
Returns: h : callable, log instance or None
The old error handler.
See also
Examples
Callback upon error:
>>> def err_handler(type, flag): ... print("Floating point error (%s), with flag %s" % (type, flag)) ...
>>> saved_handler = np.seterrcall(err_handler) >>> save_err = np.seterr(all='call')
>>> np.array([1, 2, 3]) / 0.0 Floating point error (divide by zero), with flag 1 array([ Inf, Inf, Inf])
>>> np.seterrcall(saved_handler) <function err_handler at 0x...> >>> np.seterr(**save_err) {'over': 'call', 'divide': 'call', 'invalid': 'call', 'under': 'call'}
Log error message:
>>> class Log(object): ... def write(self, msg): ... print("LOG: %s" % msg) ...
>>> log = Log() >>> saved_handler = np.seterrcall(log) >>> save_err = np.seterr(all='log')
>>> np.array([1, 2, 3]) / 0.0 LOG: Warning: divide by zero encountered in divide array([ Inf, Inf, Inf])
>>> np.seterrcall(saved_handler) <__main__.Log object at 0x...> >>> np.seterr(**save_err) {'over': 'log', 'divide': 'log', 'invalid': 'log', 'under': 'log'}
numpy.seterrcall()
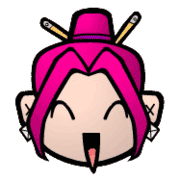
2017-01-10 18:18:39
Please login to continue.