-
numpy.linalg.svd(a, full_matrices=1, compute_uv=1)
[source] -
Singular Value Decomposition.
Factors the matrix
a
asu * np.diag(s) * v
, whereu
andv
are unitary ands
is a 1-d array ofa
?s singular values.Parameters: a : (..., M, N) array_like
A real or complex matrix of shape (
M
,N
) .full_matrices : bool, optional
If True (default),
u
andv
have the shapes (M
,M
) and (N
,N
), respectively. Otherwise, the shapes are (M
,K
) and (K
,N
), respectively, whereK
= min(M
,N
).compute_uv : bool, optional
Whether or not to compute
u
andv
in addition tos
. True by default.Returns: u : { (..., M, M), (..., M, K) } array
Unitary matrices. The actual shape depends on the value of
full_matrices
. Only returned whencompute_uv
is True.s : (..., K) array
The singular values for every matrix, sorted in descending order.
v : { (..., N, N), (..., K, N) } array
Unitary matrices. The actual shape depends on the value of
full_matrices
. Only returned whencompute_uv
is True.Raises: LinAlgError
If SVD computation does not converge.
Notes
New in version 1.8.0.
Broadcasting rules apply, see the
numpy.linalg
documentation for details.The decomposition is performed using LAPACK routine _gesdd
The SVD is commonly written as
a = U S V.H
. Thev
returned by this function isV.H
andu = U
.If
U
is a unitary matrix, it means that it satisfiesU.H = inv(U)
.The rows of
v
are the eigenvectors ofa.H a
. The columns ofu
are the eigenvectors ofa a.H
. For rowi
inv
and columni
inu
, the corresponding eigenvalue iss[i]**2
.If
a
is amatrix
object (as opposed to anndarray
), then so are all the return values.Examples
>>> a = np.random.randn(9, 6) + 1j*np.random.randn(9, 6)
Reconstruction based on full SVD:
>>> U, s, V = np.linalg.svd(a, full_matrices=True) >>> U.shape, V.shape, s.shape ((9, 9), (6, 6), (6,)) >>> S = np.zeros((9, 6), dtype=complex) >>> S[:6, :6] = np.diag(s) >>> np.allclose(a, np.dot(U, np.dot(S, V))) True
Reconstruction based on reduced SVD:
>>> U, s, V = np.linalg.svd(a, full_matrices=False) >>> U.shape, V.shape, s.shape ((9, 6), (6, 6), (6,)) >>> S = np.diag(s) >>> np.allclose(a, np.dot(U, np.dot(S, V))) True
numpy.linalg.svd()
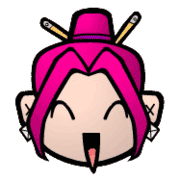
2017-01-10 18:14:49
Please login to continue.