-
numpy.ma.make_mask(m, copy=False, shrink=True, dtype=)
[source] -
Create a boolean mask from an array.
Return
m
as a boolean mask, creating a copy if necessary or requested. The function can accept any sequence that is convertible to integers, ornomask
. Does not require that contents must be 0s and 1s, values of 0 are interepreted as False, everything else as True.Parameters: m : array_like
Potential mask.
copy : bool, optional
Whether to return a copy of
m
(True) orm
itself (False).shrink : bool, optional
Whether to shrink
m
tonomask
if all its values are False.dtype : dtype, optional
Data-type of the output mask. By default, the output mask has a dtype of MaskType (bool). If the dtype is flexible, each field has a boolean dtype. This is ignored when
m
isnomask
, in which casenomask
is always returned.Returns: result : ndarray
A boolean mask derived from
m
.Examples
12345678910>>>
import
numpy.ma as ma
>>> m
=
[
True
,
False
,
True
,
True
]
>>> ma.make_mask(m)
array([
True
,
False
,
True
,
True
], dtype
=
bool
)
>>> m
=
[
1
,
0
,
1
,
1
]
>>> ma.make_mask(m)
array([
True
,
False
,
True
,
True
], dtype
=
bool
)
>>> m
=
[
1
,
0
,
2
,
-
3
]
>>> ma.make_mask(m)
array([
True
,
False
,
True
,
True
], dtype
=
bool
)
Effect of the
shrink
parameter.1234567>>> m
=
np.zeros(
4
)
>>> m
array([
0.
,
0.
,
0.
,
0.
])
>>> ma.make_mask(m)
False
>>> ma.make_mask(m, shrink
=
False
)
array([
False
,
False
,
False
,
False
], dtype
=
bool
)
Using a flexible
dtype
.12345678910111213141516>>> m
=
[
1
,
0
,
1
,
1
]
>>> n
=
[
0
,
1
,
0
,
0
]
>>> arr
=
[]
>>>
for
man, mouse
in
zip
(m, n):
... arr.append((man, mouse))
>>> arr
[(
1
,
0
), (
0
,
1
), (
1
,
0
), (
1
,
0
)]
>>> dtype
=
np.dtype({
'names'
:[
'man'
,
'mouse'
],
'formats'
:[np.
int
, np.
int
]})
>>> arr
=
np.array(arr, dtype
=
dtype)
>>> arr
array([(
1
,
0
), (
0
,
1
), (
1
,
0
), (
1
,
0
)],
dtype
=
[(
'man'
,
'<i4'
), (
'mouse'
,
'<i4'
)])
>>> ma.make_mask(arr, dtype
=
dtype)
array([(
True
,
False
), (
False
,
True
), (
True
,
False
), (
True
,
False
)],
dtype
=
[(
'man'
,
'|b1'
), (
'mouse'
,
'|b1'
)])
numpy.ma.make_mask()
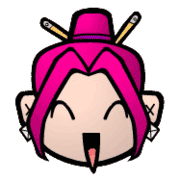
2025-01-10 15:47:30
Please login to continue.