-
numpy.ma.masked_values(x, value, rtol=1e-05, atol=1e-08, copy=True, shrink=True)
[source] -
Mask using floating point equality.
Return a MaskedArray, masked where the data in array
x
are approximately equal tovalue
, i.e. where the following condition is True(abs(x - value) <= atol+rtol*abs(value))
The fill_value is set to
value
and the mask is set tonomask
if possible. For integers, consider usingmasked_equal
.Parameters: x : array_like
Array to mask.
value : float
Masking value.
rtol : float, optional
Tolerance parameter.
atol : float, optional
Tolerance parameter (1e-8).
copy : bool, optional
Whether to return a copy of
x
.shrink : bool, optional
Whether to collapse a mask full of False to
nomask
.Returns: result : MaskedArray
The result of masking
x
where approximately equal tovalue
.See also
-
masked_where
- Mask where a condition is met.
-
masked_equal
- Mask where equal to a given value (integers).
Examples
>>> import numpy.ma as ma >>> x = np.array([1, 1.1, 2, 1.1, 3]) >>> ma.masked_values(x, 1.1) masked_array(data = [1.0 -- 2.0 -- 3.0], mask = [False True False True False], fill_value=1.1)
Note that
mask
is set tonomask
if possible.>>> ma.masked_values(x, 1.5) masked_array(data = [ 1. 1.1 2. 1.1 3. ], mask = False, fill_value=1.5)
For integers, the fill value will be different in general to the result of
masked_equal
.>>> x = np.arange(5) >>> x array([0, 1, 2, 3, 4]) >>> ma.masked_values(x, 2) masked_array(data = [0 1 -- 3 4], mask = [False False True False False], fill_value=2) >>> ma.masked_equal(x, 2) masked_array(data = [0 1 -- 3 4], mask = [False False True False False], fill_value=999999)
-
numpy.ma.masked_values()
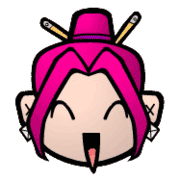
2017-01-10 18:15:36
Please login to continue.