-
numpy.ma.outerproduct(a, b)
[source] -
Compute the outer product of two vectors.
Given two vectors,
a = [a0, a1, ..., aM]
andb = [b0, b1, ..., bN]
, the outer product [R51] is:1234[[a0
*
b0 a0
*
b1 ... a0
*
bN ]
[a1
*
b0 .
[ ... .
[aM
*
b0 aM
*
bN ]]
Parameters: a : (M,) array_like
First input vector. Input is flattened if not already 1-dimensional.
b : (N,) array_like
Second input vector. Input is flattened if not already 1-dimensional.
out : (M, N) ndarray, optional
A location where the result is stored
New in version 1.9.0.
Returns: out : (M, N) ndarray
out[i, j] = a[i] * b[j]
See also
inner
,einsum
Notes
Masked values are replaced by 0.
References
[R51] (1, 2) : G. H. Golub and C. F. van Loan, Matrix Computations, 3rd ed., Baltimore, MD, Johns Hopkins University Press, 1996, pg. 8. Examples
Make a (very coarse) grid for computing a Mandelbrot set:
123456789101112131415161718192021>>> rl
=
np.outer(np.ones((
5
,)), np.linspace(
-
2
,
2
,
5
))
>>> rl
array([[
-
2.
,
-
1.
,
0.
,
1.
,
2.
],
[
-
2.
,
-
1.
,
0.
,
1.
,
2.
],
[
-
2.
,
-
1.
,
0.
,
1.
,
2.
],
[
-
2.
,
-
1.
,
0.
,
1.
,
2.
],
[
-
2.
,
-
1.
,
0.
,
1.
,
2.
]])
>>> im
=
np.outer(
1j
*
np.linspace(
2
,
-
2
,
5
), np.ones((
5
,)))
>>> im
array([[
0.
+
2.j
,
0.
+
2.j
,
0.
+
2.j
,
0.
+
2.j
,
0.
+
2.j
],
[
0.
+
1.j
,
0.
+
1.j
,
0.
+
1.j
,
0.
+
1.j
,
0.
+
1.j
],
[
0.
+
0.j
,
0.
+
0.j
,
0.
+
0.j
,
0.
+
0.j
,
0.
+
0.j
],
[
0.
-
1.j
,
0.
-
1.j
,
0.
-
1.j
,
0.
-
1.j
,
0.
-
1.j
],
[
0.
-
2.j
,
0.
-
2.j
,
0.
-
2.j
,
0.
-
2.j
,
0.
-
2.j
]])
>>> grid
=
rl
+
im
>>> grid
array([[
-
2.
+
2.j
,
-
1.
+
2.j
,
0.
+
2.j
,
1.
+
2.j
,
2.
+
2.j
],
[
-
2.
+
1.j
,
-
1.
+
1.j
,
0.
+
1.j
,
1.
+
1.j
,
2.
+
1.j
],
[
-
2.
+
0.j
,
-
1.
+
0.j
,
0.
+
0.j
,
1.
+
0.j
,
2.
+
0.j
],
[
-
2.
-
1.j
,
-
1.
-
1.j
,
0.
-
1.j
,
1.
-
1.j
,
2.
-
1.j
],
[
-
2.
-
2.j
,
-
1.
-
2.j
,
0.
-
2.j
,
1.
-
2.j
,
2.
-
2.j
]])
An example using a ?vector? of letters:
12345>>> x
=
np.array([
'a'
,
'b'
,
'c'
], dtype
=
object
)
>>> np.outer(x, [
1
,
2
,
3
])
array([[a, aa, aaa],
[b, bb, bbb],
[c, cc, ccc]], dtype
=
object
)
numpy.ma.outerproduct()
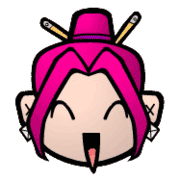
2025-01-10 15:47:30
Please login to continue.