-
numpy.where(condition[, x, y])
-
Return elements, either from
x
ory
, depending oncondition
.If only
condition
is given, returncondition.nonzero()
.Parameters: condition : array_like, bool
When True, yield
x
, otherwise yieldy
.x, y : array_like, optional
Values from which to choose.
x
andy
need to have the same shape ascondition
.Returns: out : ndarray or tuple of ndarrays
If both
x
andy
are specified, the output array contains elements ofx
wherecondition
is True, and elements fromy
elsewhere.If only
condition
is given, return the tuplecondition.nonzero()
, the indices wherecondition
is True.Notes
If
x
andy
are given and input arrays are 1-D,where
is equivalent to:[xv if c else yv for (c,xv,yv) in zip(condition,x,y)]
Examples
>>> np.where([[True, False], [True, True]], ... [[1, 2], [3, 4]], ... [[9, 8], [7, 6]]) array([[1, 8], [3, 4]])
>>> np.where([[0, 1], [1, 0]]) (array([0, 1]), array([1, 0]))
>>> x = np.arange(9.).reshape(3, 3) >>> np.where( x > 5 ) (array([2, 2, 2]), array([0, 1, 2])) >>> x[np.where( x > 3.0 )] # Note: result is 1D. array([ 4., 5., 6., 7., 8.]) >>> np.where(x < 5, x, -1) # Note: broadcasting. array([[ 0., 1., 2.], [ 3., 4., -1.], [-1., -1., -1.]])
Find the indices of elements of
x
that are ingoodvalues
.>>> goodvalues = [3, 4, 7] >>> ix = np.in1d(x.ravel(), goodvalues).reshape(x.shape) >>> ix array([[False, False, False], [ True, True, False], [False, True, False]], dtype=bool) >>> np.where(ix) (array([1, 1, 2]), array([0, 1, 1]))
numpy.where()
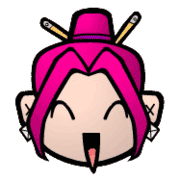
2017-01-10 18:19:13
Please login to continue.