-
numpy.delete(arr, obj, axis=None)
[source] -
Return a new array with sub-arrays along an axis deleted. For a one dimensional array, this returns those entries not returned by
arr[obj]
.Parameters: arr : array_like
Input array.
obj : slice, int or array of ints
Indicate which sub-arrays to remove.
axis : int, optional
The axis along which to delete the subarray defined by
obj
. Ifaxis
is None,obj
is applied to the flattened array.Returns: out : ndarray
A copy of
arr
with the elements specified byobj
removed. Note thatdelete
does not occur in-place. Ifaxis
is None,out
is a flattened array.Notes
Often it is preferable to use a boolean mask. For example:
>>> mask = np.ones(len(arr), dtype=bool) >>> mask[[0,2,4]] = False >>> result = arr[mask,...]
Is equivalent to
np.delete(arr, [0,2,4], axis=0)
, but allows further use ofmask
.Examples
>>> arr = np.array([[1,2,3,4], [5,6,7,8], [9,10,11,12]]) >>> arr array([[ 1, 2, 3, 4], [ 5, 6, 7, 8], [ 9, 10, 11, 12]]) >>> np.delete(arr, 1, 0) array([[ 1, 2, 3, 4], [ 9, 10, 11, 12]])
>>> np.delete(arr, np.s_[::2], 1) array([[ 2, 4], [ 6, 8], [10, 12]]) >>> np.delete(arr, [1,3,5], None) array([ 1, 3, 5, 7, 8, 9, 10, 11, 12])
numpy.delete()
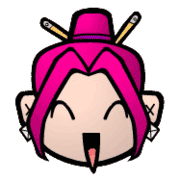
2017-01-10 18:13:37
Please login to continue.