-
numpy.insert(arr, obj, values, axis=None)
[source] -
Insert values along the given axis before the given indices.
Parameters: arr : array_like
Input array.
obj : int, slice or sequence of ints
Object that defines the index or indices before which
values
is inserted.New in version 1.8.0.
Support for multiple insertions when
obj
is a single scalar or a sequence with one element (similar to calling insert multiple times).values : array_like
Values to insert into
arr
. If the type ofvalues
is different from that ofarr
,values
is converted to the type ofarr
.values
should be shaped so thatarr[...,obj,...] = values
is legal.axis : int, optional
Axis along which to insert
values
. Ifaxis
is None thenarr
is flattened first.Returns: out : ndarray
A copy of
arr
withvalues
inserted. Note thatinsert
does not occur in-place: a new array is returned. Ifaxis
is None,out
is a flattened array.See also
-
append
- Append elements at the end of an array.
-
concatenate
- Join a sequence of arrays along an existing axis.
-
delete
- Delete elements from an array.
Notes
Note that for higher dimensional inserts
obj=0
behaves very different fromobj=[0]
just likearr[:,0,:] = values
is different fromarr[:,[0],:] = values
.Examples
>>> a = np.array([[1, 1], [2, 2], [3, 3]]) >>> a array([[1, 1], [2, 2], [3, 3]]) >>> np.insert(a, 1, 5) array([1, 5, 1, 2, 2, 3, 3]) >>> np.insert(a, 1, 5, axis=1) array([[1, 5, 1], [2, 5, 2], [3, 5, 3]])
Difference between sequence and scalars:
>>> np.insert(a, [1], [[1],[2],[3]], axis=1) array([[1, 1, 1], [2, 2, 2], [3, 3, 3]]) >>> np.array_equal(np.insert(a, 1, [1, 2, 3], axis=1), ... np.insert(a, [1], [[1],[2],[3]], axis=1)) True
>>> b = a.flatten() >>> b array([1, 1, 2, 2, 3, 3]) >>> np.insert(b, [2, 2], [5, 6]) array([1, 1, 5, 6, 2, 2, 3, 3])
>>> np.insert(b, slice(2, 4), [5, 6]) array([1, 1, 5, 2, 6, 2, 3, 3])
>>> np.insert(b, [2, 2], [7.13, False]) # type casting array([1, 1, 7, 0, 2, 2, 3, 3])
>>> x = np.arange(8).reshape(2, 4) >>> idx = (1, 3) >>> np.insert(x, idx, 999, axis=1) array([[ 0, 999, 1, 2, 999, 3], [ 4, 999, 5, 6, 999, 7]])
-
numpy.insert()
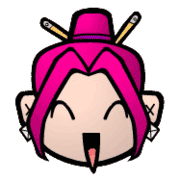
2025-01-10 15:47:30
Please login to continue.