-
numpy.linalg.matrix_power(M, n)
[source] -
Raise a square matrix to the (integer) power
n
.For positive integers
n
, the power is computed by repeated matrix squarings and matrix multiplications. Ifn == 0
, the identity matrix of the same shape as M is returned. Ifn < 0
, the inverse is computed and then raised to theabs(n)
.Parameters: M : ndarray or matrix object
Matrix to be ?powered.? Must be square, i.e.
M.shape == (m, m)
, withm
a positive integer.n : int
The exponent can be any integer or long integer, positive, negative, or zero.
Returns: M**n : ndarray or matrix object
The return value is the same shape and type as
M
; if the exponent is positive or zero then the type of the elements is the same as those ofM
. If the exponent is negative the elements are floating-point.Raises: LinAlgError
If the matrix is not numerically invertible.
See also
-
matrix
- Provides an equivalent function as the exponentiation operator (
**
, not^
).
Examples
>>> from numpy import linalg as LA >>> i = np.array([[0, 1], [-1, 0]]) # matrix equiv. of the imaginary unit >>> LA.matrix_power(i, 3) # should = -i array([[ 0, -1], [ 1, 0]]) >>> LA.matrix_power(np.matrix(i), 3) # matrix arg returns matrix matrix([[ 0, -1], [ 1, 0]]) >>> LA.matrix_power(i, 0) array([[1, 0], [0, 1]]) >>> LA.matrix_power(i, -3) # should = 1/(-i) = i, but w/ f.p. elements array([[ 0., 1.], [-1., 0.]])
Somewhat more sophisticated example
>>> q = np.zeros((4, 4)) >>> q[0:2, 0:2] = -i >>> q[2:4, 2:4] = i >>> q # one of the three quarternion units not equal to 1 array([[ 0., -1., 0., 0.], [ 1., 0., 0., 0.], [ 0., 0., 0., 1.], [ 0., 0., -1., 0.]]) >>> LA.matrix_power(q, 2) # = -np.eye(4) array([[-1., 0., 0., 0.], [ 0., -1., 0., 0.], [ 0., 0., -1., 0.], [ 0., 0., 0., -1.]])
-
numpy.linalg.matrix_power()
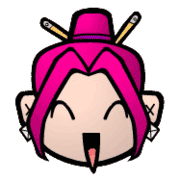
2017-01-10 18:14:46
Please login to continue.