-
numpy.piecewise(x, condlist, funclist, *args, **kw)
[source] -
Evaluate a piecewise-defined function.
Given a set of conditions and corresponding functions, evaluate each function on the input data wherever its condition is true.
Parameters: x : ndarray
The input domain.
condlist : list of bool arrays
Each boolean array corresponds to a function in
funclist
. Wherevercondlist[i]
is True,funclist[i](x)
is used as the output value.Each boolean array in
condlist
selects a piece ofx
, and should therefore be of the same shape asx
.The length of
condlist
must correspond to that offunclist
. If one extra function is given, i.e. iflen(funclist) - len(condlist) == 1
, then that extra function is the default value, used wherever all conditions are false.funclist : list of callables, f(x,*args,**kw), or scalars
Each function is evaluated over
x
wherever its corresponding condition is True. It should take an array as input and give an array or a scalar value as output. If, instead of a callable, a scalar is provided then a constant function (lambda x: scalar
) is assumed.args : tuple, optional
Any further arguments given to
piecewise
are passed to the functions upon execution, i.e., if calledpiecewise(..., ..., 1, 'a')
, then each function is called asf(x, 1, 'a')
.kw : dict, optional
Keyword arguments used in calling
piecewise
are passed to the functions upon execution, i.e., if calledpiecewise(..., ..., lambda=1)
, then each function is called asf(x, lambda=1)
.Returns: out : ndarray
The output is the same shape and type as x and is found by calling the functions in
funclist
on the appropriate portions ofx
, as defined by the boolean arrays incondlist
. Portions not covered by any condition have a default value of 0.Notes
This is similar to choose or select, except that functions are evaluated on elements of
x
that satisfy the corresponding condition fromcondlist
.The result is:
123456|
-
-
|funclist[
0
](x[condlist[
0
]])
out
=
|funclist[
1
](x[condlist[
1
]])
|...
|funclist[n2](x[condlist[n2]])
|
-
-
Examples
Define the sigma function, which is -1 for
x < 0
and +1 forx >= 0
.123>>> x
=
np.linspace(
-
2.5
,
2.5
,
6
)
>>> np.piecewise(x, [x <
0
, x >
=
0
], [
-
1
,
1
])
array([
-
1.
,
-
1.
,
-
1.
,
1.
,
1.
,
1.
])
Define the absolute value, which is
-x
forx <0
andx
forx >= 0
.12>>> np.piecewise(x, [x <
0
, x >
=
0
], [
lambda
x:
-
x,
lambda
x: x])
array([
2.5
,
1.5
,
0.5
,
0.5
,
1.5
,
2.5
])
numpy.piecewise()
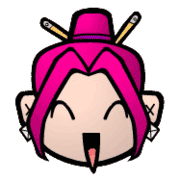
2025-01-10 15:47:30
Please login to continue.