-
numpy.ma.masked_object(x, value, copy=True, shrink=True)
[source] -
Mask the array
x
where the data are exactly equal to value.This function is similar to
masked_values
, but only suitable for object arrays: for floating point, usemasked_values
instead.Parameters: x : array_like
Array to mask
value : object
Comparison value
copy : {True, False}, optional
Whether to return a copy of
x
.shrink : {True, False}, optional
Whether to collapse a mask full of False to nomask
Returns: result : MaskedArray
The result of masking
x
where equal tovalue
.See also
-
masked_where
- Mask where a condition is met.
-
masked_equal
- Mask where equal to a given value (integers).
-
masked_values
- Mask using floating point equality.
Examples
>>> import numpy.ma as ma >>> food = np.array(['green_eggs', 'ham'], dtype=object) >>> # don't eat spoiled food >>> eat = ma.masked_object(food, 'green_eggs') >>> print(eat) [-- ham] >>> # plain ol` ham is boring >>> fresh_food = np.array(['cheese', 'ham', 'pineapple'], dtype=object) >>> eat = ma.masked_object(fresh_food, 'green_eggs') >>> print(eat) [cheese ham pineapple]
Note that
mask
is set tonomask
if possible.>>> eat masked_array(data = [cheese ham pineapple], mask = False, fill_value=?)
-
numpy.ma.masked_object()
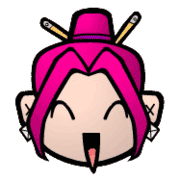
2017-01-10 18:15:36
Please login to continue.