-
class sklearn.model_selection.PredefinedSplit(test_fold)
[source] -
Predefined split cross-validator
Splits the data into training/test set folds according to a predefined scheme. Each sample can be assigned to at most one test set fold, as specified by the user through the
test_fold
parameter.Read more in the User Guide.
Examples
>>> from sklearn.model_selection import PredefinedSplit >>> X = np.array([[1, 2], [3, 4], [1, 2], [3, 4]]) >>> y = np.array([0, 0, 1, 1]) >>> test_fold = [0, 1, -1, 1] >>> ps = PredefinedSplit(test_fold) >>> ps.get_n_splits() 2 >>> print(ps) PredefinedSplit(test_fold=array([ 0, 1, -1, 1])) >>> for train_index, test_index in ps.split(): ... print("TRAIN:", train_index, "TEST:", test_index) ... X_train, X_test = X[train_index], X[test_index] ... y_train, y_test = y[train_index], y[test_index] TRAIN: [1 2 3] TEST: [0] TRAIN: [0 2] TEST: [1 3]
Methods
get_n_splits
([X, y, groups])Returns the number of splitting iterations in the cross-validator split
([X, y, groups])Generate indices to split data into training and test set. -
__init__(test_fold)
[source]
-
get_n_splits(X=None, y=None, groups=None)
[source] -
Returns the number of splitting iterations in the cross-validator
Parameters: X : object
Always ignored, exists for compatibility.
y : object
Always ignored, exists for compatibility.
groups : object
Always ignored, exists for compatibility.
Returns: n_splits : int
Returns the number of splitting iterations in the cross-validator.
-
split(X=None, y=None, groups=None)
[source] -
Generate indices to split data into training and test set.
Parameters: X : object
Always ignored, exists for compatibility.
y : object
Always ignored, exists for compatibility.
groups : object
Always ignored, exists for compatibility.
Returns: train : ndarray
The training set indices for that split.
test : ndarray
The testing set indices for that split.
-
model_selection.PredefinedSplit()
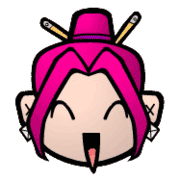
2017-01-15 04:24:16
Please login to continue.