-
class numpy.errstate(**kwargs)
[source] -
Context manager for floating-point error handling.
Using an instance of
errstate
as a context manager allows statements in that context to execute with a known error handling behavior. Upon entering the context the error handling is set withseterr
andseterrcall
, and upon exiting it is reset to what it was before.Parameters: kwargs : {divide, over, under, invalid}
Keyword arguments. The valid keywords are the possible floating-point exceptions. Each keyword should have a string value that defines the treatment for the particular error. Possible values are {?ignore?, ?warn?, ?raise?, ?call?, ?print?, ?log?}.
See also
Notes
The
with
statement was introduced in Python 2.5, and can only be used there by importing it:from __future__ import with_statement
. In earlier Python versions thewith
statement is not available.For complete documentation of the types of floating-point exceptions and treatment options, see
seterr
.Examples
>>> from __future__ import with_statement # use 'with' in Python 2.5 >>> olderr = np.seterr(all='ignore') # Set error handling to known state.
>>> np.arange(3) / 0. array([ NaN, Inf, Inf]) >>> with np.errstate(divide='warn'): ... np.arange(3) / 0. ... __main__:2: RuntimeWarning: divide by zero encountered in divide array([ NaN, Inf, Inf])
>>> np.sqrt(-1) nan >>> with np.errstate(invalid='raise'): ... np.sqrt(-1) Traceback (most recent call last): File "<stdin>", line 2, in <module> FloatingPointError: invalid value encountered in sqrt
Outside the context the error handling behavior has not changed:
>>> np.geterr() {'over': 'warn', 'divide': 'warn', 'invalid': 'warn', 'under': 'ignore'}
numpy.errstate()
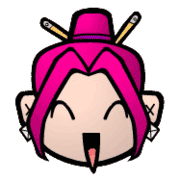
2017-01-10 18:13:47
Please login to continue.